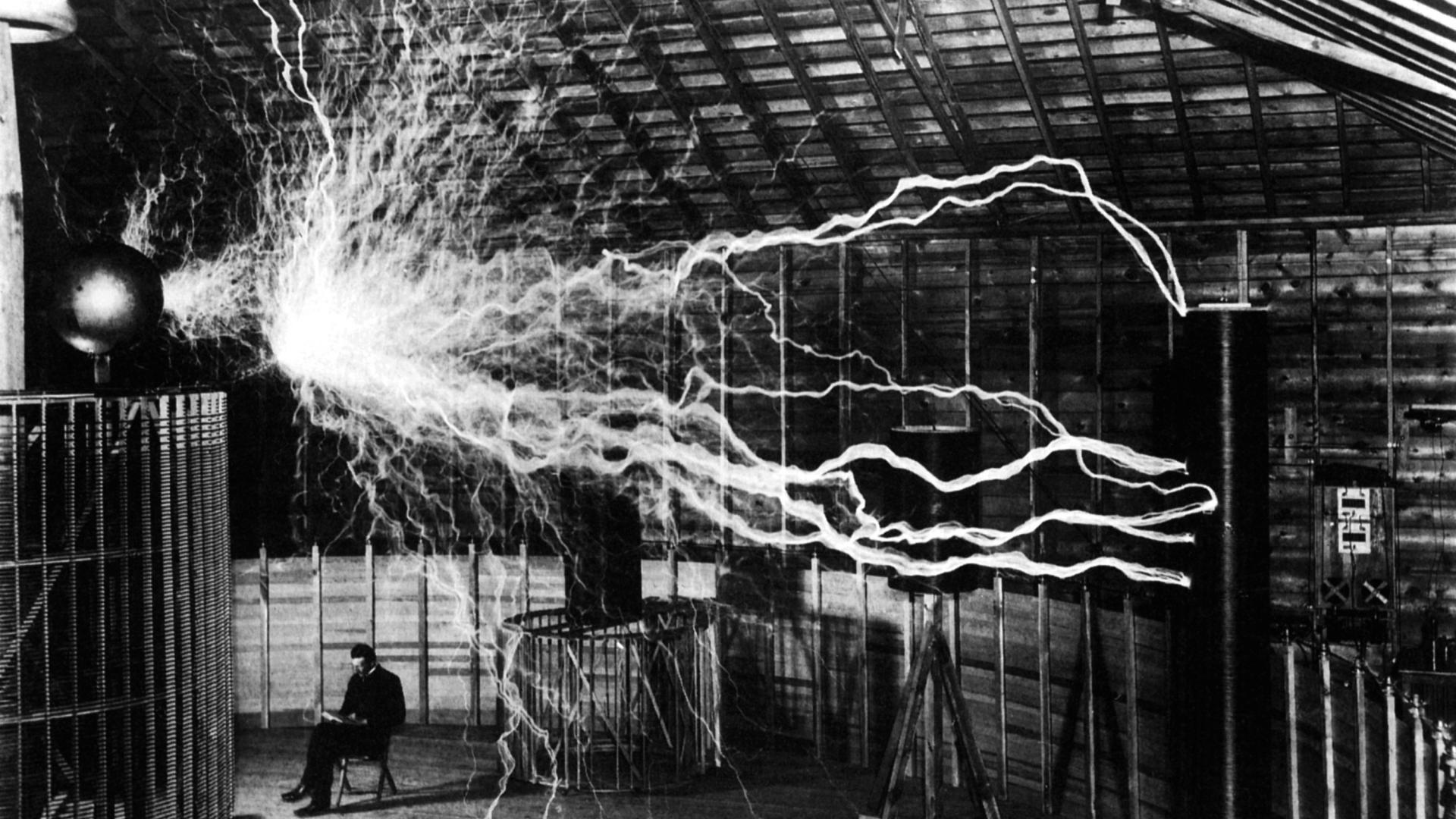
Introduction to jQuery Guide · Dynamic Web Sites
JavaScript is a great scripting language and is very fast, however, due to its simplicity and differences in browsers, it can be a bit unwieldy to tackle some common front end tasks. jQuery is a JavaScript library of expressive, cross-browser, convenience methods. jQuery is great for:
- Searching and iterating over page elements
- Modifying pages’ HTML structure (aka the DOM)
- Listening/ responding to events
- AJAX’ing data to/ from the server
- Animating page content
The most important function in jQuery is called $ which is shorthand for the jQuery function.
$( “selector-string-here”) selects an element or group of elements that match the selector string and returns them in jQuery array format which can be operated on or iterated over.
Selecting Elements:
jQuery DOM iteration or manipulation usually starts with selecting the relevant page elements:
To select all page elements (* operator):
var els = $('*');
To select all elements with a given tag (just provide the tag name):
var els = $('h1'); var els = $('p'); var els = $('div');
To select all elements with a given class (. operator):
var els = $('.myClass');
The :not() Operator (Select elements that do not match selector):
I am raw html block.
Click edit button to change this html
var els = $(':not(.myClass)'); //All elements that don't have the class myClass
To select the element with a given id (# operator):
var els = $('#elemId');
To select all elements with a given attribute:
var els = $('[href]'); //Selects all elements with href attribute set var els = $('[src]'); //Selects all elements with src attribute set
To select all link elements with a certain href attribute:
var els = $('a[href="/home"]'); //Selects all link elements with href of /home
Combo Selectors:
Selectors can be combined to further filter the returned elements
To select all elements with a given tag that have a certain class:
var els = $('h1.myClass');
To select all elements with two classes:
var els = $('.myClass.myOtherClass');
To select all elements with a class and pseudoclass:
var els = $('.myClass:visible ');
Use commas to combine multiple selectors and match on any of the selectors (OR):
var els = $('h1, h2, h3');
Descendent Operators
Selectors can be combined with descendent operators (space or >) to further filter the returned elements. This is by no means a comprehensive collection of jQuery selectors. The important takeaway is the syntax of basic selectors and how selectors can be combined to fine tune which elements are targeted.
Children selectors: the > operator means to select direct children descendants
To select all children of the element with id=elemId:
var els = $('#elemId > *');
To select all children of the element with id=elemId that have a certain class:
var els = $('#elemId > .myClass');
To select all of the #elemId element’s children links with a certain href attribute:
var els = $('#elemId > a[href="/home"]');
Descendent selectors: the space (” “) operator means to select all descendants
To select all descendants of the element with id=elemId:
var els = $('#elemId *');
To select all descendants of the element with id=elemId that have a certain class:
var els = $('#elemId .myClass');
To select all descendants links with a certain href attribute:
var els = $('#elemId a[href="/home"]');
Pseudoclass selectors:
var els = $('input[type="checkbox"]:checked'); //All checked boxes var els = $('input:focus'); //Focused input field (ie blinking cursor) var els = $(''input:visible'); //All visible inputs
Iterating Over Elements
There are two ways to iterate over the DOM elements returned from a jQuery selection. In both cases, you’ll need to wrap the element in $(element) to perform additional jQuery functions:
els.each(function() { var domEl = this; var jqEl = $(domEl); //Do something here });
for(var i = 0; i < els.length; i++) { var domEl = els[i]; var jqEl = $(domEl); //Do something here });
Accessing Element Details
jQuery provides many convenient ways to access elements’ classes, attributes, sizing & styles:
var jqEl = $("#elemId"); jqEl.hasClass("myClass"); //true or false jqEl.attr("myAttribute"); //String value of attribute or null if not set jqEl.is(":visible"); //true or false jqEl.is(":focus"); //true or false jqEl.is("#elemId"); //true or false jqEl.width(); //Width of element in pixels jqEl.height(); //Width of element in pixels jqEl.html(); //Gets the inner html of the element jqEl.val(); //For form elements, gets the entered value jqEl.css("background-color"); //Gets the background-color css rule jqEl.offset(); // top and left offsets, for ex: {top: 100px, left: 100px}
Traversing
Once you’ve selected an element you may want to find its’ ancestor elements or descendent elements
var jqEl = $("#elemId"); var parentEl = jqEl.parent(); var closestAnscEl = jqEl.closest('.myClass'); //Closest ancestor with myClass class var childrenEls = jqEl.children(); //All children var descEls = jqEl.find("*"); //All descendants var childrenElsWithClass = jqEl.find(".myClass"); //All descendants with myClass class
Operating Upon Elements
jqEl.addClass("myClass"); //Ensures element has myClass class jqEl.removeClass("myClass"); //Removes myClass class from element, if it exists jqEl.width(100); //Change element width to 100px jqEl.height(100); //Change element height to 100px jqEl.css("background-color", "green"); //Apply a CSS property setting jqEl.attr("href", "/home"); //Set attribute jqEl.removeAttr("href"); //Removes attribute jqEl.prop("checked", true); //Check a checkbox, (false unchecks) jqEl.append("Some extra content"); //Add some extra content to the end of the element jqEl.prepend("Some extra content"); //Add some extra content to the beginning of the element jqEl.after("Some extra content
"); //Add some extra content directly after the element jqEl.before("Some extra content
"); //Add some extra content directly before the element
Showing/ Hiding/ Removing Elements
jqEl.hide(); //Makes the element hidden jqEl.show(); //Makes the element visible jqEl.fadeOut(); //Fades the element to hidden jqEl.fadeIn(); //Fades the element to visible jqEl.remove(); //Deletes the element from the page
Chaining jQuery Functions
jQuery was written so that most of its functions return a jQuery element selection so that you can chain together calls:
$("#elemId").parent().parent().find('.myClass').css("color ", "blue").fadeIn();
Remote Data/ Interacting With The Server (AJAX)
jQuery has a nice cross-browser solution for AJAX calls:
$.ajax( url:"path/to/server/script", data: { propA:"Value a", propB:"Value b" }, cache:false, method:"POST" ).done(function(msg) { //Often times, you'll need to parse the JSON response to an object var responseObj = JSON.parse(msg); });
Waiting for the Page to Load: The $(document).ready function
$(document).ready(function() { //Handle the event });
jQuery Event Listeners: The $(document).on function
jQuery has an easy interface for responding to events, the $(document).on function:
$(document).on(events, selector, function(event) { //Handle the event });
$(document).on Parameter Explanation:
Events: string representing which event to listen for. To listen for multiple events, separate with spaces.
Selector: the string that represents which elements for which to listen for these events
The Handler Function: The third parameter to .on is the handler function. There is some useful information that you can leverage in the handler function:
$(document).on("click", "#elElem", function(event) { //Element that triggered event, will match selector this //The element that was interacted with (HTML Element) $(this) //The element that was interacted with (jQuery Element) //Actual element that the user interacted with (could be a child of this, or this itself) event.target //The element that user interacted with (HTML element) $(event.target) //The element that user interacted with (jQuery element) //Where the click occurred: page will be >= client because page includes visible portion as //well as any scrolling. Client is only inclusive of the visible portion event.pageX //Pixels from the very leftmost edge of the page event.pageY //Pixels from the very topmost edge of the page event.clientX //Pixels from the leftmost edge of the visible portion of page event.clientY //Pixels from the topmost edge of the visible portion of page //Available event actions: event.preventDefault(); //Cancel default action });
A common confusion is the difference between this and event.target. this will always be the element that was interacted with and will match the selector(2nd parameter in $(document).on). event.target is even more precise and may be a descendent of this or this itself.
For example, imagine you have a button with an icon
and a click handler installed with $(document).on(“click”, “.btn”, function(event) { … });
In the case when the user clicks directly on the button’s icon, this will be the button element and event.target will be the icon element.
jQuery Plugins
jQuery has a great community which has created some amazing open source plugins:
jQuery UI
Datatables
Pickadate
Chosen
…and many more…
In-Browser Developer Tools
One of the best ways to debug scripts or develop new JavaScript or jQuery code is to open up your developer console in IE, Chrome, or Firefox. This is as easy as pressing the F12 key. There are a couple of interesting sections which each have a tab to navigate between:
Elements: View the HTML of the page in real time. Similar to “view source” except dynamic and shows the current working copy of the page
Console: View any programmatic output from calls to console.log() and run JavaScript(and jQuery) in real time, in the context of the page (or debug breakpoint)
Sources: Browse any loaded scripts and add breakpoints so that you can step through the program line by line
Network: See all resources that are loaded as well as the responses. Great for seeing the XHR/ AJAX calls parameters and responses
Application: See all of the site’s cookie data
Elements:
To quickly find an element on the page, click on the inspect button and then click on the page element:
The Styles section under Elements is a great way to see which CSS rules are being applied to the element and makes it easy to try out and refine new CSS in real time. Select the element you want to style in the Elements section and tweak the styles as necessary.
For example, clicking on the swatch next to a color property opens a nice color selector widget to help you find the perfect color:
To add a new CSS property, click to the right of the last CSS property:
Console:
To try out new JavaScript or jQuery code, type it in the console and hit enter.
Note: To add a new line without executing the code, press shift+enter
Sources:
To delve into the source code for a page, or to do interactive debugging, go to the sources tab. To debug, find the relevant portion of the code and add a breakpoint by clicking on the line number of where you would like to pause execution:
The next time program execution reaches that line in the javascript, the program will pause and the line will highlight blue:
On the left side, you will see a directory listing of all the loaded files that assemble the page.
On the right side, you will see the call stack and can click to navigate. Also, the Scope section will show all the variables that are currently in scope. Importantly, the debug controls are in the top right of the sources section:
In order, the buttons are:
Resume script execution- when your done with this portion of the code, click this and the code will continue running until the next breakpoint reached
Step over (go to the next line of code) – Most used
Step into next function call- if the highlighted line consists of a function call, you can use this option to goto that function to walkthrough its execution
Step out of next function call- if you are currently walking through a function and you want to jump back to where the function was called, press this button. Also, the Call Stack section will show you where you are in nested function calls
In recent browser versions, variable values are indicated to the right of each line when in debug mode. Mousing over a variable name will also show a tooltip with its value.
When the browser is in debug mode, you can switch to the console tab and try out code in the context of this portion of code.
Network:
The network section gives you details about loaded resources and XHR calls. To view the response text, click on the request in the list and select “Response” tab:
To view the content as a JSON object with collapse/ expand options for nested values
To see which information is sent to the server with the request, click on the “Headers” tab
Search All Files:
Often times, we’ll need to track down code relating to a certain element or variable but we don’t know exactly which file or where in the file to look. Chrome has a feature called Search All Files that does a cross file search of all the loaded resources:
Click the three vertical dots on the top right of dev tools:
Select Search All Files
At the bottom of dev tools you’ll see the search bar and search results