- Home
- JavaScript
- Getting Started With JavaScript
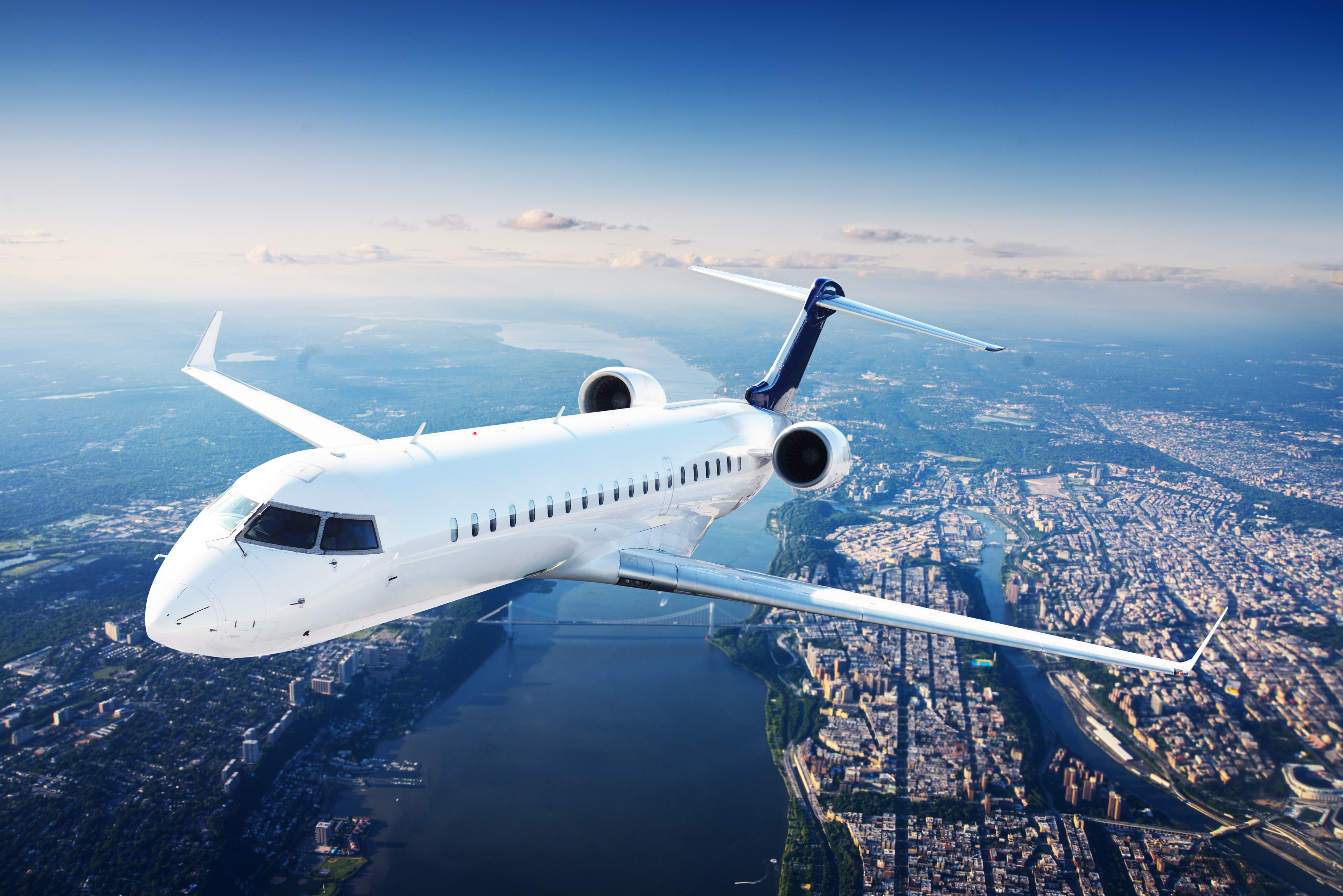
Getting Started With JavaScript
JavaScript is great for running additional logic after the page is loaded by Coldfusion. JavaScript is a scripting language for dynamic page content and runs in the user browser’s memory, freeing the server’s valuable resources.
Script Location in HTML
Most all JavaScript lives within <script> tags, usually placed in the <head> of the html document
<script type=”text/javascript”>
…Javascript Code Goes Here…
</script>
Alternatively, you can define your JavaScript in a separate file(often given .js file extension) and include the file with the script tag’s src attribute:
<script type=”text/javascript” src=”path/to/js/file.js”></script>
JavaScript Variables:
JavaScript variables are the building blocks of data. Open up your browser’s dev tools console and run the following code to try it out. Not sure what dev tools are or what the console is? More info is included at the end of this guide)
Initialization
All JavaScript variables are initialized with the var keyword:
var favNum = 7;
Once initialized, variables are referenced by name(without the var keyword):
favNum++;
console.log(favNum); //Prints 8 to the console
Variable Types
JavaScript supports numerous variable types. The developer never has to explicitly specify which type the variable will have, you just set it to the appropriate value. The typeof operator returns the type for a given variable:
var myBool = true;
var myNum = 5;
var myString = ‘Hello’; //Single or double quotes both permitted by JavaScript for strings
var myArray =[true, 5, ‘Hello’];
var myObject ={fName: ‘Robert’, lName: ‘Testerson’};
Even functions can be stored to a variable, allowing you to pass callback functions as parameters to other functions for execution later
var myAlertMsgFunct = function(msg) {
alert(“The message is: ” + msg);
};
myAlertMsgFunct(“Trust Authority”); //Alerts “The message is: Trust Authority”
You can retrieve the type for a variable with the typeof operator:
console.log(typeof myBool); //Prints “boolean”
console.log(typeof myNum); //Prints “number”
console.log(typeof myString); //Prints “string”
console.log(typeof myArray); //Prints “object”
console.log(typeof myObject); //Prints “object”
console.log(typeof myAlertMsgFunct); //Prints “function”
JavaScript Booleans
The standard way to initialize a Boolean:
var myBool = false;
Boolean variables can also be initialized to the result of a condition:
var myBool = (10 == 10);
Checking Boolean equality is done with either the == or === operators:
if(myBool == true) { console.log(“They’re equal”); }
if(myBool === true) { console.log(“They’re equal”); }
Difference between == and ===:
0 == false (evaluates to true)
0 === false (evaluates to false)
1 == true (evaluates to true)
1 === true (evaluates to false)
JavaScript Numbers
JavaScript supports int and float numbers:
var a = 5;
var b = 5.5;
Adding, subtracting, multiplying, and dividing numbers are done with the +, -, *, and / operators respectfully:
var c = a+b;
var d = a-b;
var e = a*b;
var f = a/b;
To convert from a string to a number, use parseInt or parseFloat
var aStr = “5”;
var a = parseInt(aStr); //a=5
var bStr = “5.5”;
var b = parseFloat(bStr); //b=5.5
JavaScript Strings
Adding strings is done with the + operator:
var fullName = firstName + ” ” + lastName;
Checking string equality is done with == operator:
if(str1 == str2) { console.log(“They’re equal”); }
Checking string irrespective of case is done with .toLower()
if(“Saturday”.toLowerCase() == “saturday”.toLowerCase()) { console.log(“They’re equal”); }
//Prints “They’re equal”;
Strings can be defined using either single or double quotes:
“Monday” == ‘Monday ‘ //Evaluates to true
JavaScript Arrays
Arrays in JavaScript allow for lists of other variables and/or values.
An empty array is initialized as follows:
var myArray = [];
Alternatively, an array can be initialized with pre-set items:
var months = [null, ‘Jan’, ‘Feb’, ‘Mar’, ‘Apr’, ‘May’, ‘Jun’, ‘Jul’, ‘Aug’, ‘Sep’, ‘Oct’, ‘Nov’, ‘Dec’];
You can access an array’s element at a certain index with ray[i]:
console.log(months[4]); //Prints “Apr”
Getting the size/length of array:
console.log(months.length); //Prints 13
You find the index of the first occurrence of a value with .indexOf(val), returns -1 if not found
months.indexOf(“Apr”); // 4
months.indexOf(“XYZ”); // -1
You can add an element to the end of an array with .push()
myArray.push(“NewElem”);
You can add an element to the beginning of an array with .unshift()
myArray.unshift(“NewElem”);
You can retrieve and remove the last element with .pop()
var lastEl = myArray.pop();
You can create an array from a string with str.split(delimiter):
myArray = “A sentence to be split into words”.split(” “);
JavaScript Objects
Objects in JavaScript allow for associative sets of keys=>values. Keys are also known as properties
An empty object is initialized as follows:
var myObj = {};
Alternatively, an object can be initialized with pre-set key/values:
var myObj = {
fName:”Suzy”,
lName:”Test”
};
There are two ways to set or override a value for a property:
myObj.mName = ‘Middle’;
or
myObj[“mName”] = ‘Middle’; //Use this approach if the property name is a script variable
Check if a property is set for an object:
myObj.hasOwnProperty(“myProp”) //true or false
Iterating over objects properties:
for(var prop in myObj) {
if(myObj.hasOwnProperty(prop)) {
console.log(“myObj.” + prop + ” = ” + myObj.prop);
}
}
There are two ways to delete an object’s property:
delete myObj.mName;
delete myObj[“mName”];
JavaScript Functions
JavaScript functions encapsulate common functionality for use throughout the program. Initializing a function involves naming it and specifying which parameters it accepts, as well as the JavaScript that should be run when called, and optionally, a return value.
function countWords(sentenceStr) {
return sentenceStr.split(” “).length;
}
var sentence = “I will support and defend the Constitution of the United States against all enemies, foreign and domestic”;
var numWords = countWords(sentence); //17
Closures & Variable Scope
A closure in JavaScript is a block of code that is contained within curly braces { } Closures are used for functions, loops and if/else conditions
Variables that are created outside of all closures are called global variables and are available throughout the program.
var myGlobalVar = “accessible everywhere”;
function myFunction() {
console.log(myGlobalVar); //Prints: “accessible everywhere”
}
myFunction();
Variables initialized within a closure are restricted in scope to that closure alone.
function myFunction() {
var myLocalVar = “accessible in closure only”;
}
myFunction();
console.log(myLocalVar); //Prints: undefined
To create a global variable, you can also set a property on the window object variable:
function myFunction() {
window.myGlobalVar = “accessible everywhere”;
}
myFunction();
console.log(myGlobalVar); //Prints: “accessible everywhere”